In this guide, we’ll delve into the practicalities of using the git revert
command—a tool that developers frequently utilize to rectify past errors without losing work. Fundamentally, the
function produces an “equal but opposite” commit, effectively neutralizing the impact of a specific commit or group of commits. This approach to reversing mistakes is often safer and more efficient than using Git reset, which might remove or orphan commits in the commit history.git revert
Throughout this article, we’ll explore the process of implementing a
in various environments—be it the terminal, GitKraken Client, or GitLens for VS Code. We’ll also introduce additional Git commands and workflows that developers can leverage to undo errors, offering insights on how to select the appropriate command based on your individual use case.git revert
offers several advantages, making it an essential tool for any developer working with Git:git revert
- It provides a safe and effective means of undoing mistakes without the risk of losing work.
- It doesn’t erase or orphan commits in the commit history.
is a forward-moving undo operation, implying that it doesn’t affect future commits.git revert
- Even for beginners,
is user-friendly and straightforward to use.git revert
As a developer utilizing Git, understanding how to execute a
is a valuable skill. It can assist you in preventing work loss and maintaining a clean and well-organized codebase. git revert
Take a look at these additional Git commands and workflows developers use to undo mistakes and how to determine which command to use based on your use case.
How to Perform a Git Revert Commit Using a Terminal
How to Perform a Git Revert Commit Using GitKraken Client
How to Perform a Git Revert Commit Using GitLens for VS Code
Whether you prefer to use a GUI or CLI, GitKraken Client offers the best of both worlds and makes reverting commits faster, easier, and safer by giving you more control.
Revert Git Commit in the Terminal
To undo a Git commit using a terminal, you’ll first need to identify the unique commit ID or SHA of the commit you want to undo. To find the commit ID for your targeted commit, run the following:
git log
This will show you a list of your commits along with each commit’s unique ID. Next, copy the commit ID of the commit you want to revert.
Now run git revert <commit ID>
. This creates a new commit that negates the commit you specified.
Git Revert Multiple Commits Using a Terminal
You can also revert a range of Git commits. To do this run the following:
git revert <older commit ID>..<newer commit ID>
The older commit should come first, followed by the newer commit. This will revert these two commits, plus any commit between them.
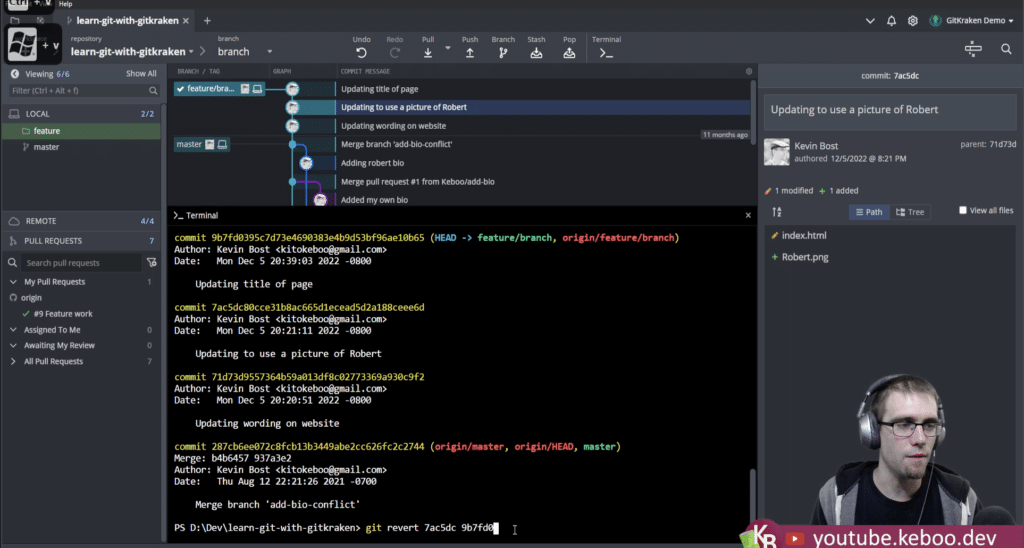
Git Revert Commit Using GitKraken Client
Reverting a Git commit can be done in just 2 clicks with the helpful visual context of GitKraken Client.
To revert a commit using GitKraken Client, simply right-click on the commit you want to revert from the central graph and select Revert commit from the context menu.
You will then be asked if you want to immediately commit the changes; from here you can choose to save the reverted commit, or select No to make additional code changes or change the Git commit message.
Pretty simple right? No need to remember complicated commit IDs or go through extra steps. Ready to try it out? Download GitKraken Client and test just how easy it is to revert a Git commit.
Git Revert Multiple Commits Using GitKraken Client
If you’re reverting more than one commit in GitKraken Client, you will need to revert them one at a time, and you should do so in order of newest to oldest. This will decrease the chance of introducing a conflict.
Git Revert Commit Using GitLens
If you’re a VS Code user, GitLens makes it easy to revert commits.
To revert a Git commit using GitLens complete the following:
- Open your repo in VS Code
- From the sidebar select Source Control
- Navigate to the COMMITS section
- Right-click on the commit you want to revert
- Select the Revert Commit option
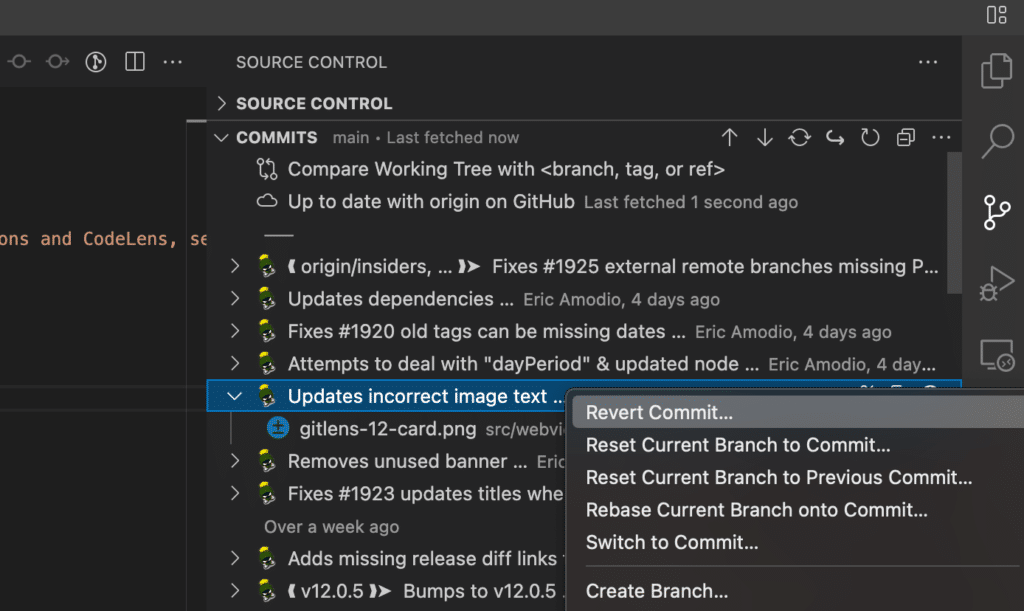
You can also access this option through the command palette by typing >GitLens: Git Revert
or from the commit details’ quick pick menu.
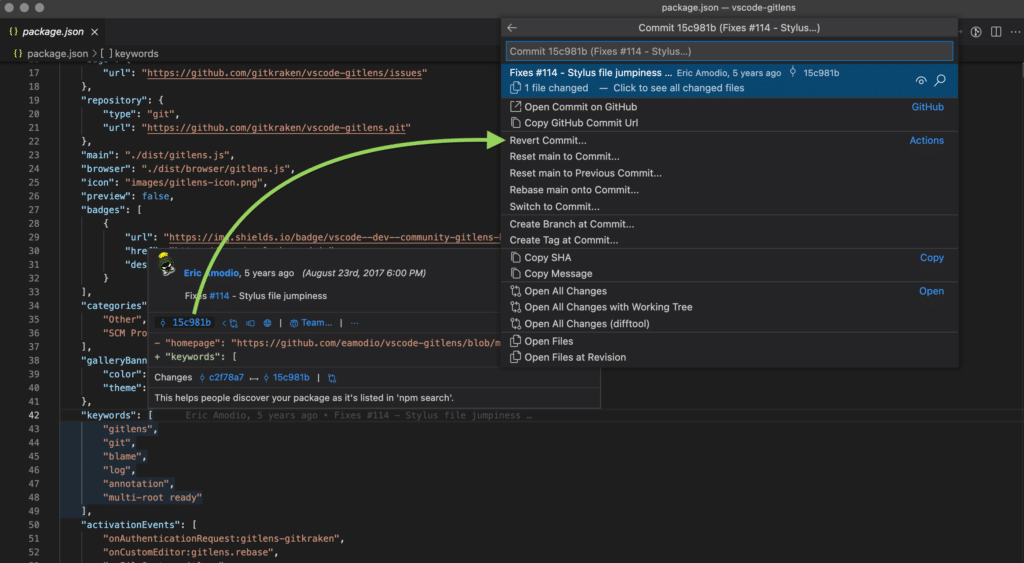
Ready to give it a try? Install GitLens to stay organized and on track with your tasks and projects.
Additional Commands and Workflows to Undo a Commit
Even the most diligent of developers run into mistakes from time to time when working with Git repositories. But the process of fixing these mistakes can differ depending on the circumstances of each case. Furthermore, if you aren’t careful with how you undo these errors, you could end up losing work or messing up the work of your team members.
Thankfully, Git offers several tools that allow you to undo mistakes introduced by a commit. As with any other toolbox, it’s important to understand the purpose, advantages, and related risks of every tool before using them.
So let’s take a look at some other common Git commands developers use to undo their mistakes.
Quick Undo Using GitKraken Client
GitKraken Client makes it easy to undo/redo the following actions using the undo and redo buttons in the top Toolbar:
- Checkout
- Commit
- Discard
- Delete branch
- Remove remote
- Reset branch to a commit
It’s important to note that the GitKraken Client undo button will only undo your most recent Git action. Undoing anything later than your most recent Git action will require the use of either Git revert, Git reset, or Git rebase.
Git Reset
While Git revert uses forward change to undo commits, the operation of Git reset is just the opposite.
Git reset is a way to move back in time to a particular commit, and to reset your active position to the chosen commit in a branch’s commit history.
However, just as science fiction movies depict, there are all sorts of side effects that can come from altering the course of history. For example, if you travel back to a commit with a reset, all the commits you passed may enter a dangling state where they exist but nothing references them. Furthermore, if you perform a “hard” reset, you can lose local work that wasn’t backed up properly.
If you need to make revisions to a commit that is not your last commit, the best solution is to create a new commit by reverting the old commit. As a Git best practice, you should avoid doing anything that will require you to force push — and rewrite the history — of your main branch(es).
Still determined to perform a Git reset? Here’s a guide to help you Git reset with as few issues as possible.
Git Rebase
Now, let’s say you want to undo a Git commit by going back in time, but you don’t want to completely reset history.
Rather than abandoning the commits after the erroneous commit, you want to apply them again and deal with the impacts of your changed history commit by commit.
For those who want a bit more manual control over the history revision process, Git provides the interactive rebase tool. With interactive rebase, you are presented with a list of commits up to a specified point on your branch’s history. From this point, you have various options for each commit:
- Pick: you can keep the commit as-is in the history
- Drop: remove the commit from the history
- Squash: combine the commit with the one before it
- Reword: change the commit message
Get step-by-step instructions on how to perform a Git rebase.
Git Amend
If you happen to catch a mistake immediately after you commit, you can quickly undo the error using the Git amend command. Perhaps you forgot to stage a file in the last commit, or had a typo in the commit message, or even made mistakes in the code, but have not yet committed anything else on top of it.
If you want to modify the last commit in your history, you have the ability to amend the Git commit with changes. For example, you can amend the previous commit by changing the Git commit message or description, or you can even stage a file you forgot to include.
Similarly, if there is a mistake in your previous commit’s code, you can fix the mistake and even add more changes before you amend it. Git amend is a single-commit undo tool which only affects the last commit in the branch’s history.
As with Git reset and Git rebase, amending is a history-rewriting process, or a reverse change, rather than a forward change. Under the hood, Git amend creates a brand new commit and replaces your last commit with the new one. It should therefore receive the same level of caution; once you have pushed a commit into a shared branch, amending that commit and pushing the amended change can result in side effects for anyone working off of that commit.
The Best Way to Change the Future
Undoing Git commits is a lot like modern depictions of time travel, with each Git command allowing you to change and affect history in a unique way. It’s up to you to determine which command fits your use case and apply it from there. Happy traveling!
Let’s do a final recap.
Git Revert
- Does NOT rewrite your repos history
- Neutralizes the effects of a specified commit or commit(s)
- Usually the best command to use to “undo” a command when working with collaborators
Git Reset
- Resets your active position to the chosen commit potentially deleting all commits that came after
- Requires you to use a Git force push
- Can cause problems for your teammates
Git Rebase
- Essentially moves commits around and keeps some of your commit history
- Using the Interactive rebase tool you can manually select what you want to do with each commit including pick, drop, squash, reword
Git Amend
- “History-rewriting” command
- Can only be performed on your latest commit
- Can be used to change a commit message, add a file, adjust code, etc.
GitKraken Client makes the process of reverting a Git commit simple and with far less risk. Do your workflow a favor and try GitKraken Client today.